Latest10 Most Popular Python Code Snippets Every Developer Should Know
In this article, we'll explore 10 of the most popular Python code snippets every developer should know.
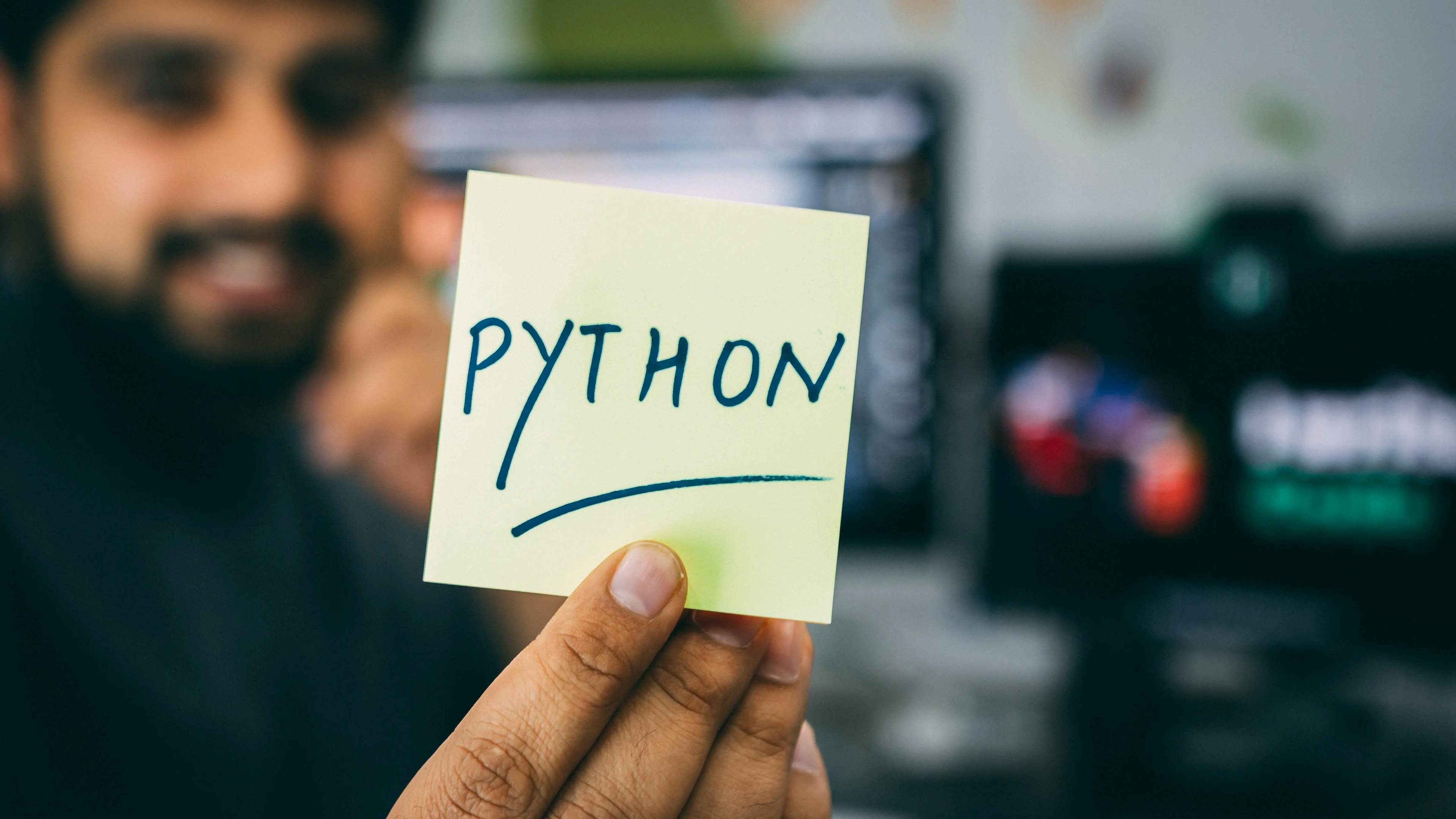
The 10 Most Popular Python Code Snippets
Welcome to the Code Snippets AI blog! Today, we're diving into the world of Python, one of the most popular programming languages. We'll be sharing the top 10 Python code snippets that our users love. Let's get started!
1. Hello, World!
print("Hello, World!")
2. Generate a list in a single line of code
squares = [x**2 for x in range(10)]
3. Efficiently read a file with Python
with open('file.txt', 'r') as file:
data = file.read()
4. Writing to a File
with open('file.txt', 'w') as file:
file.write("Hello, Code Snippets AI!")
5. Define a simple function in Python
def greet(name):
return f"Hello, {name}!"
6. Lambda Function
multiply = lambda x, y: x * y
7. Error Handling
try:
# risky code
except Exception as e:
print(f"Caught an exception: {e}")
8. Dictionary Comprehension
squares = {x: x**2 for x in range(10)}
9. Define a class and create an object
class MyClass:
x = 5
p1 = MyClass()
print(p1.x)
10. Import a Module
import math
By mastering these 10 Python code snippets, you'll be able to streamline your coding process and write more efficient and effective code. Whether you're a beginner or an experienced developer, these snippets are essential tools to have in your coding arsenal.
Ready for the next step? Level up your coding skills today with Code Snippets AI